Design Circular Queue
Get started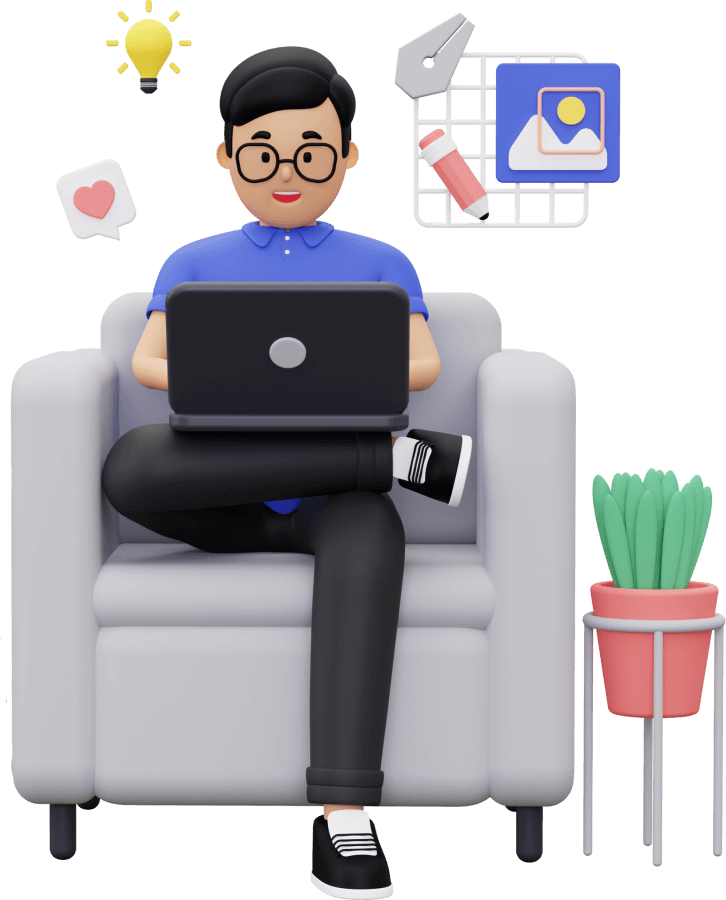
জয় শ্রী রাম
🕉.gif)
Problem Statement:
Design your implementation of the circular queue. The circular queue is a linear data structure in which the operations are performed based on FIFO (First In First Out) principle and the last position is connected back to the first position to make a circle. It is also called "Ring Buffer".One of the benefits of the circular queue is that we can make use of the spaces in front of the queue. In a normal queue, once the queue becomes full, we cannot insert the next element even if there is a space in front of the queue. But using the circular queue, we can use the space to store new values.
Implement the CircularQueue class:
Front(): gets the front item from the queue. If the queue is empty, return -1.
Rear(): gets the last item from the queue. If the queue is empty, return -1.
enQueue(int value): inserts an element into the circular queue. Return true if the operation is successful.
deQueue(): deletes an element from the circular queue. Return true if the operation is successful.
isEmpty(): checks whether the circular queue is empty or not.
isFull(): checks whether the circular queue is full or not. The circular queue should have a pre-set maximum capacity and should not contain more
You must solve the problem without using the built-in queue data structure in your programming language.
Java and Python Solution:
Low Level Design:
Login to Access Content
Is your implementation thread-safe ? If not, how would you make it thread-safe ?
Login to Access Content