Generate Parentheses
Get started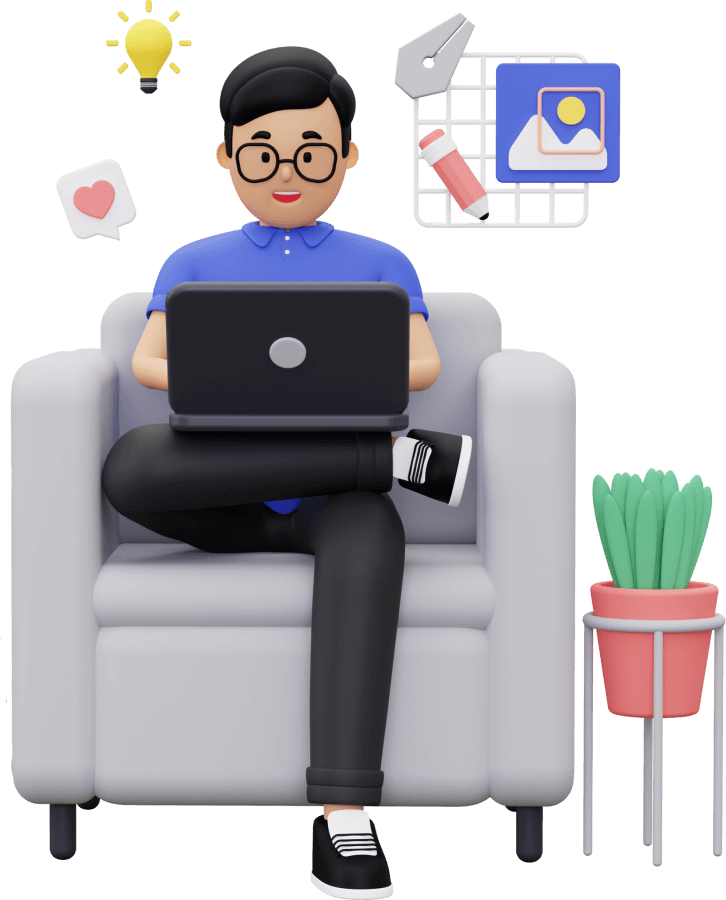
জয় শ্রী রাম
🕉Problem Statement:
Given n pairs of parentheses, write a function to generate all combinations of well-formed parentheses.
Example 1:
Input: n = 3
Output: ["((()))","(()())","(())()","()(())","()()()"]
Example 2:
Input: n = 1
Output: ["()"]
Solution:
- NOTE: I highly recommend going through the Backtracking chapters in the order they are given in the Index page to get the most out of it and be able to build a rock-solid understanding.
If you are familiar with our backtracking template then you would be easily come up with a quick solution for this problem just by making the below two observations:
We need to ask ourselves two questions:
- When can we add an opening brace "(" to a given empty or non-empty partial solution ?
- When can we add a closing brace ")" to a given empty or non-empty partial solution ?
Looking at the patterns carefully, we can conclude:
-
We always have room for appending one more opening brace "("
to a given partial solution
as long as partial solution contains less than n opening braces.
"(" can be added to an empty partial solution.
Keep in mind we cannot have more than n opening braces in our solution.
-
We can append closing brace ")" to our partial solution
only when we have less number of closing braces than opening braces in our current partial solution.
")" cannot be added to an empty partial solution.
Java Code:
Login to Access Content
Python Code:
Login to Access Content
Time Complexity:
Please subscribe to access the complexity analysis.
Don't forget to take in-depth look at the other backtracking problems because that is what would make you comfortable with using the backtracking template and master the art of Backtracking:
- Letter Case Permutation
- Power Set
- All Paths Between Two Nodes
- Word Search
- Sudoku
- N-Queens
- Word Square